In the previous tutorial we took a first look at MySQL and Phpmyadmin. In this video we will run our first MySQL Query through PHP to create a table inside of our …
Original source
PHP Tutorial 27 – MySQL Creating A Table (PHP For Beginners)
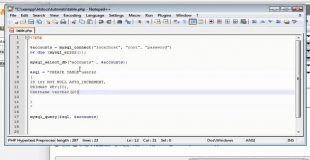
In the previous tutorial we took a first look at MySQL and Phpmyadmin. In this video we will run our first MySQL Query through PHP to create a table inside of our …
Original source
34 responses to “PHP Tutorial 27 – MySQL Creating A Table (PHP For Beginners)”
// php code to create table on mysql
<?php
$servername = "localhost";
$username = "root"; //type your username
$password = "**"; //type your password
$dbname = "accounts"; /type your database name
// Create connection
$conn = new mysqli($servername, $username, $password, $dbname);
//mysqli stands for my scripted query language improve.
// Check connection
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
// sql to create table
$sql = "CREATE TABLE MyGuests (
id INT(6) UNSIGNED AUTO_INCREMENT PRIMARY KEY,
firstname VARCHAR(30) NOT NULL,
lastname VARCHAR(30) NOT NULL,
email VARCHAR(50),
reg_date TIMESTAMP
)";
if ($conn->query($sql) === TRUE) {
echo "Table accounts created successfully";
} else {
echo "Error creating table: " . $conn->error;
}
$conn->close();
?>
/ to create database on mysql using php code
<?php
$servername="localhost";
$username="root"; /type your username
$password="***"; /type your password
$conn=new mysqli("$servername","$username","$password");
if($conn->connect_error)
{
die("not connected".$conn->connect_error);
}
$sql="CREATE DATABASE MYdb";
if($conn->query($sql)=== true)
{
echo"database created";
}
else
{
echo"not created".$conn->error;
}
$conn->close();
?>
I am not able to find table in my database and i dont find any errors in my php code
Warning: mysql_select_db() expects parameter 2 to be resource
How to fix this?
useless nothing working even with mysqli
mine only worked with mysqli_connect, it gave me this error message:
( ! )
Deprecated: mysql_connect(): The mysql extension is deprecated and will
be removed in the future: use mysqli or PDO instead in
C:wampwwwtestmysql.php on line 8
very helping..thnx chris
para-meter? don't you mean pa-rameter?
First video to not start with "hello youtube!" you've changed Chris..
It works.. thanks a lot
why did you quit making videos ? you were great
i m gettin a syntax error on line 9 its bcuz of the bracket (
Doesn't work for me
I had no errors…. and no table….
It works :
<?php
$con = mysqli_connect("localhost","root","","accounts1");
// Check connection
// Create table
$sql = "CREATE TABLE users7
(
ID int NOT NULL AUTO_INCREMENT,
PRIMARY KEY(ID),
Username varchar(20),
Password varchar(20),
FirstName varchar(20),
LastName varchar(20)
)";
// Execute query
mysqli_query($con,$sql)
I have watched all his videos, and actually made a page that allows you to make an account then login in to view the information, but now it wont let me access the mysql database, without changing any of the code, i am unable to access it. I can still use php, and XAMPP shows now errors with Mysql. i used the code from when i first watched this video and it still does not allow me to access the database
Mines didn't make a user2 table and I called $sql="CREATE TABLE user2"
i'd encountered this error, Parse error: syntax error, unexpected '$sql' (T_VARIABLE)
It's actually sad that I've learned more in less than a day from this guy than half a year at a university in UK.
my man……here is my code…..gives no errors on the localhost, but does not table 'users 2' does not show up on sql
<?php
$accounts = mysql_connect("localhost" , "root" , "Baseball82")
or die (mysql_error());
mysql_select_db("accounts" , $accounts);
$sql = "CREATE TABLE users2
(
ID int NOT NULL AUTO_INCREMENT,
PRIMARY KEY(ID),
Username varchar(20),
Password varchar(20),
First varchar(20),
Last varchar(20),
)
";
mysql_query($sql, $accounts);
thanks dude
Hi! I have a problem! I created a .php file and checked him and it's seems nothing wrong but, on MyAdmin there is no my table that I created. When I try to import table MySQL reports me an error, but i couldn't find it. Code seems me ok. Here is the code:
<?php
$konekcija = mysql_connect ("localhost" , "root", "26081990") or die (mysql_error());
mysql_select_db("nalog", $konekcija);
$tabela = "CREATE TABLE ljudi
(
ID int NOT NULL AUTO_INCREMENT,
PRIMARY KEY(ID),
Korisnicko_ime varchar(20),
Sifra varchar(20),
Ime varchar(20),
Prezime(20)
)
";
mysql_query($tabela, $konekcija);
?>
an this is the error that MySQL reports me:]
Error
MySQL said: Documentation
#1064 – You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '<?php
$konekcija = mysql_connect ("localhost" , "root", "26081990") or die (' at line 1
If anyone has a solution please answer me. THANK YOU!
He said ' ' with this ones you can concatenate with the . then you contacatenate the variables but " " you cannot do it.
Why use " " now and on previous 20 tutorials ' ' ? I've seen differences you've mentioned in one of the tutorials but I still don't understand. Thanks in advance!
hello, good day, this video is very great. My I ask if you have any latest update video tutorial? Hope you can create more videos about mysql. Thanks
I see a lot of people having problems with the code in the tut as did I. To solve this problem remove the comma from the last field.
Hey Chris, I would request you to upload some video tutorials of javascript as well.
Chris, your tutorials are great. I'm trying to create the table.php page but I'm not working with a "localhost". I'm saving the files to my website on a shared server. Can you tell me what should replace "localhost"? –Cheers
Why can't I create the new table with the same code in the video? My code is below:
<?php
$accounts = mysql_connect("localhost", "root", "zaqxsw") or die(mysql_error());
mysql_select_db("accounts", $accounts);
$sql = "CREATE TABLE users2
(
ID Int NOT NULL AUTO_INCREMENT,
PRIMARY KEY(ID),
Username varchar(20),
Password varchar(20),
First varchar(20),
Last varchar(20)
)
";
mysql_query ($sql, $accounts);
?>
Booot! xD
Congratulations!
if your having problems with it creating the second table, make sure you are not putting any spaces in the name of row properties, so "first name varchar(20)" would be "firstname varchar(20)"
hey dud @suresh kumar, change the curly braces in CREATE TABLE instead use parenthesis.. surely u'll get
have a nce day
<?php
$accounts= mysql_connect("localhost","root","janaki") or die(mysql_error());
mysql_select_db("database " , $accounts);
$sql= "CREATE TABLE example3
{
ID Int AUTO_INCREMENT NOT NULL,
PRIMARY KEY(ID),
username varchar(20),
password varchar(20),
firstname varchar(20),
lastname varchar(20)
}";
mysql_query($sql,$accounts);
?>
could you pls tell me whats wrong on this code, no error throws, but table is not created
If you are having trouble, try this:
<?php
$con = mysqli_connect("localhost","root","password","accounts");
// Check connection
if (mysqli_connect_errno())
{
echo "Failed to connect to MySQL: " . mysqli_connect_error();
}
// Create table
$sql = "CREATE TABLE users2
(
ID int NOT NULL AUTO_INCREMENT,
PRIMARY KEY(ID),
Username varchar(20),
Password varchar(20),
FirstName varchar(20),
LastName varchar(20)
)";
// Execute query
if (mysqli_query($con,$sql))
{
echo "Table created successfully";
}
else
{
echo "Error creating table: " . mysqli_error($con);
}
?>